728x90
반응형
📃버튼을 클릭하면 다른 액티비티로 이동하시오.
📝layout1.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:padding="20dp">
<TextView
android:id="@+id/tv_info"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="버튼을 누르면 입력 액티비티를 실행합니다."
android:textSize="20sp" />
<Button
android:id="@+id/btn"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="입력창 실행"
android:textSize="25sp" />
<TextView
android:id="@+id/tv"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:gravity="center"
android:textSize="30sp" />
</LinearLayout>
📝layout2.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<EditText
android:id="@+id/et"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
<LinearLayout
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:orientation="horizontal"
android:layout_marginLeft="200dp">
<Button
android:id="@+id/btn_change"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="CHANGE" />
<Button
android:id="@+id/btn_cancel"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="CANCEL" />
</LinearLayout>
</LinearLayout>
📝MainActivity.java
package com.example.test02;
import androidx.annotation.Nullable;
import androidx.appcompat.app.AppCompatActivity;
import android.content.Intent;
import android.os.Bundle;
import android.util.Log;
import android.view.View;
import android.widget.EditText;
public class MainActivity extends AppCompatActivity {
final int REQUEST_CODE_START_INPUT = 1;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.layout1);
findViewById(R.id.btn).setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Intent intent = new Intent(MainActivity.this, MainActivity2.class);
startActivity(intent);
}
});
}
}
📝MainActivity2.java
package com.example.test02;
import androidx.appcompat.app.AppCompatActivity;
import android.content.Intent;
import android.os.Bundle;
import android.view.View;
import android.widget.EditText;
public class MainActivity2 extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.layout2);
findViewById(R.id.btn_change).setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
EditText et = (EditText) findViewById(R.id.et);
Intent intent = new Intent();
intent.putExtra("input", et.getText().toString()+"");
setResult(RESULT_OK, intent);
finish();
}
});
findViewById(R.id.btn_cancel).setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
finish();
}
});
}
}
📃EditText의 text값이 Log에 출력되도록 하시오.
📝MainActivity.java
package com.example.test02;
import androidx.annotation.Nullable;
import androidx.appcompat.app.AppCompatActivity;
import android.content.Intent;
import android.os.Bundle;
import android.util.Log;
import android.view.View;
import android.widget.EditText;
public class MainActivity extends AppCompatActivity {
final int REQUEST_CODE_START_INPUT = 1;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.layout1);
findViewById(R.id.btn).setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Intent intent = new Intent(MainActivity.this, MainActivity2.class);
startActivityForResult(intent, REQUEST_CODE_START_INPUT);
}
});
}
@Override
protected void onActivityResult(int requestCode, int resultCode, @Nullable Intent data) {
super.onActivityResult(requestCode, resultCode, data);
Log.i("jyeon", "onActivityResult");
if(resultCode == RESULT_OK) {
String str = data.getStringExtra("input");
Log.i("jyeon", "onActivityResult : " + str);
}
}
}
📝MainActivity2.java
package com.example.test02;
import androidx.appcompat.app.AppCompatActivity;
import android.content.Intent;
import android.os.Bundle;
import android.view.View;
import android.widget.EditText;
public class MainActivity2 extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.layout2);
findViewById(R.id.btn_change).setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
EditText et = (EditText) findViewById(R.id.et);
Intent intent = new Intent();
intent.putExtra("input", et.getText().toString()+"");
setResult(RESULT_OK, intent);
finish();
}
});
findViewById(R.id.btn_cancel).setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
finish();
}
});
}
}
📃추가적으로, EditText에 입력한 값이 첫 번째 액티비티 화면의 TextView에도 출력되게 하시오.


📝MainActivity.java
package com.example.test02;
import androidx.annotation.Nullable;
import androidx.appcompat.app.AppCompatActivity;
import android.content.Intent;
import android.os.Bundle;
import android.util.Log;
import android.view.View;
import android.widget.EditText;
import android.widget.TextView;
public class MainActivity extends AppCompatActivity {
final int REQUEST_CODE_START_INPUT = 1;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.layout1);
findViewById(R.id.btn).setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Intent intent = new Intent(MainActivity.this, MainActivity2.class);
startActivityForResult(intent, REQUEST_CODE_START_INPUT);
}
});
}
@Override
protected void onActivityResult(int requestCode, int resultCode, @Nullable Intent data) {
super.onActivityResult(requestCode, resultCode, data);
Log.i("jyeon", "onActivityResult");
TextView tv = findViewById(R.id.tv);
if(resultCode == RESULT_OK) {
String str = data.getStringExtra("input");
tv.setText(str+"을/를 입력하셨습니다.");
}
}
}
📝MainActivity.java
package com.example.test02;
import androidx.appcompat.app.AppCompatActivity;
import android.content.Intent;
import android.os.Bundle;
import android.view.View;
import android.widget.EditText;
public class MainActivity2 extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.layout2);
findViewById(R.id.btn_change).setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
EditText et = (EditText) findViewById(R.id.et);
Intent intent = new Intent();
intent.putExtra("input", et.getText().toString()+"");
setResult(RESULT_OK, intent);
finish();
}
});
findViewById(R.id.btn_cancel).setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
finish();
}
});
}
}
◾ onActivityResult 메소드의 파라미터가 의미하는 것들
📃리스트뷰의 아이템을 누르면 다음 액티비티로 이동하고, 텍스트뷰에 있는 텍스트를 Log에 출력하시오.
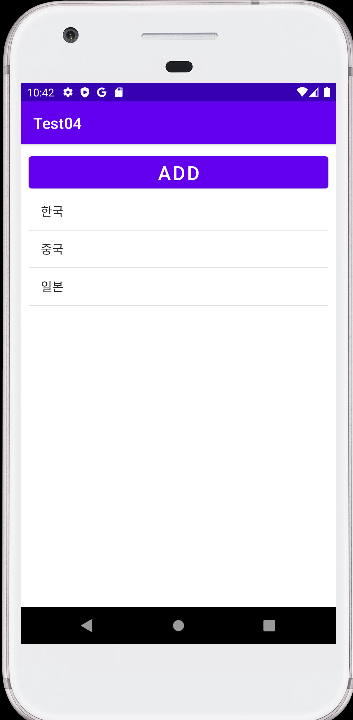

📝layout1.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:padding="10dp">
<Button
android:id="@+id/btn_add"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="add"
android:textSize="25sp" />
<ListView
android:id="@+id/lv"
android:layout_width="match_parent"
android:layout_height="match_parent" />
</LinearLayout>
📝layout2.xml
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:padding="20dp">
<EditText
android:id="@+id/et_input"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:textSize="30sp" />
<Button
android:id="@+id/btn_ok"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@id/et_input"
android:layout_alignParentRight="true"
android:text="OK"
android:textSize="20sp" />
<Button
android:id="@+id/btn_cancel"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@id/et_input"
android:layout_marginRight="20dp"
android:layout_toLeftOf="@id/btn_ok"
android:text="cancel"
android:textSize="20sp" />
</RelativeLayout>
📝MainActivity.java
package com.example.test04;
import androidx.annotation.Nullable;
import androidx.appcompat.app.AppCompatActivity;
import android.content.Intent;
import android.os.Bundle;
import android.view.View;
import android.widget.EditText;
public class MainActivity2 extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.layout2);
EditText et_input = findViewById(R.id.et_input);
et_input.setText(getIntent().getStringExtra("data"));
findViewById(R.id.btn_ok).setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Intent intent = getIntent();
EditText et_input = findViewById(R.id.et_input);
String str = et_input.getText().toString();
intent = intent.putExtra("input", str+"");
setResult(RESULT_OK, intent);
finish();
}
});
findViewById(R.id.btn_cancel).setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
finish();
}
});
}
}
📝MainActivity2.java
package com.example.test02;
import androidx.appcompat.app.AppCompatActivity;
import android.content.Intent;
import android.os.Bundle;
import android.view.View;
import android.widget.EditText;
public class MainActivity2 extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.layout2);
findViewById(R.id.btn_change).setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
EditText et = (EditText) findViewById(R.id.et);
Intent intent = new Intent();
intent.putExtra("input", et.getText().toString()+"");
setResult(RESULT_OK, intent);
finish();
}
});
findViewById(R.id.btn_cancel).setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
finish();
}
});
}
}


📝MainActivity.java
package com.example.test04;
import androidx.annotation.Nullable;
import androidx.appcompat.app.AppCompatActivity;
import android.content.Intent;
import android.os.Bundle;
import android.util.Log;
import android.view.View;
import android.widget.AdapterView;
import android.widget.ArrayAdapter;
import android.widget.ListView;
import java.util.ArrayList;
public class MainActivity extends AppCompatActivity {
ArrayList<String> al = new ArrayList<>();
ArrayAdapter<String> aa;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.layout1);
al.add("한국");
al.add("중국");
al.add("일본");
aa = new ArrayAdapter<>(this, android.R.layout.simple_list_item_1, al);
ListView lv = findViewById(R.id.lv);
lv.setAdapter(aa);
lv.setOnItemClickListener(lis);
}
AdapterView.OnItemClickListener lis = new AdapterView.OnItemClickListener() {
@Override
public void onItemClick(AdapterView<?> parent, View view, int position, long id) {
Intent intent = new Intent(MainActivity.this, MainActivity2.class);
intent.putExtra("data", al.get(position)+"");
intent.putExtra("index", position); // 클릭한 아이템의 해당 인덱스를 저장한다.
startActivityForResult(intent, 1);
}
};
@Override
protected void onActivityResult(int requestCode, int resultCode, @Nullable Intent data) {
super.onActivityResult(requestCode, resultCode, data);
if(resultCode == RESULT_OK) {
if(requestCode == 1) {
String str = data.getStringExtra("result");
Log.i("jyeon", str + "");
int index = data.getIntExtra("index", -1);
al.set(index, str);
aa.notifyDataSetChanged();
}
}
}
}
📝MainActivity2.java
package com.example.test04;
import androidx.annotation.Nullable;
import androidx.appcompat.app.AppCompatActivity;
import android.content.Intent;
import android.os.Bundle;
import android.view.View;
import android.widget.EditText;
public class MainActivity2 extends AppCompatActivity {
Intent myOrgIntent;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.layout2);
EditText et_input = findViewById(R.id.et_input);
et_input.setText(getIntent().getStringExtra("data"));
findViewById(R.id.btn_ok).setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
myOrgIntent = getIntent();
EditText et_input = findViewById(R.id.et_input);
String str = et_input.getText().toString();
myOrgIntent.putExtra("result", str+"");
setResult(RESULT_OK, myOrgIntent);
finish();
}
});
findViewById(R.id.btn_cancel).setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
finish();
}
});
}
}
📃ADD 버튼 누르면 리스트뷰 아이템을 추가하시오.

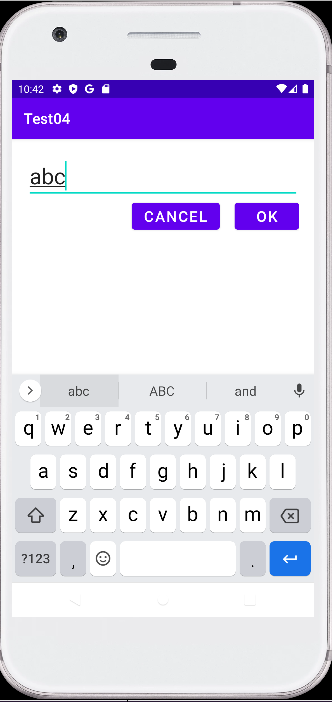
📝MainActivity.java
package com.example.test04;
import androidx.annotation.Nullable;
import androidx.appcompat.app.AppCompatActivity;
import android.content.Intent;
import android.os.Bundle;
import android.util.Log;
import android.view.View;
import android.widget.AdapterView;
import android.widget.ArrayAdapter;
import android.widget.ListView;
import java.util.ArrayList;
public class MainActivity extends AppCompatActivity {
ArrayList<String> al = new ArrayList<>();
ArrayAdapter<String> aa;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.layout1);
al.add("한국");
al.add("중국");
al.add("일본");
aa = new ArrayAdapter<>(this, android.R.layout.simple_list_item_1, al);
ListView lv = findViewById(R.id.lv);
lv.setAdapter(aa);
lv.setOnItemClickListener(lis);
findViewById(R.id.btn_add).setOnClickListener(lis_btn);
}
View.OnClickListener lis_btn = new View.OnClickListener() {
@Override
public void onClick(View v) {
Intent i = new Intent(MainActivity.this, MainActivity2.class);
startActivityForResult(i, 2);
}
};
AdapterView.OnItemClickListener lis = new AdapterView.OnItemClickListener() {
@Override
public void onItemClick(AdapterView<?> parent, View view, int position, long id) {
Intent intent = new Intent(MainActivity.this, MainActivity2.class);
intent.putExtra("data", al.get(position)+"");
intent.putExtra("index", position); // 클릭한 아이템의 해당 인덱스를 저장한다.
startActivityForResult(intent, 1);
}
};
@Override
protected void onActivityResult(int requestCode, int resultCode, @Nullable Intent data) {
super.onActivityResult(requestCode, resultCode, data);
if(resultCode == RESULT_OK) {
if(requestCode == 1) {
String str = data.getStringExtra("result");
Log.i("jyeon", str + "");
int index = data.getIntExtra("index", -1);
al.set(index, str);
aa.notifyDataSetChanged();
}
else if(requestCode == 2) {
String str = data.getStringExtra("result");
al.add(str);
aa.notifyDataSetChanged();
}
}
}
}
📝MainActivity2.java
package com.example.test04;
import androidx.annotation.Nullable;
import androidx.appcompat.app.AppCompatActivity;
import android.content.Intent;
import android.os.Bundle;
import android.view.View;
import android.widget.EditText;
public class MainActivity2 extends AppCompatActivity {
Intent myOrgIntent;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.layout2);
EditText et_input = findViewById(R.id.et_input);
et_input.setText(getIntent().getStringExtra("data"));
findViewById(R.id.btn_ok).setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
myOrgIntent = getIntent();
EditText et_input = findViewById(R.id.et_input);
String str = et_input.getText().toString();
myOrgIntent.putExtra("result", str+"");
setResult(RESULT_OK, myOrgIntent);
finish();
}
});
findViewById(R.id.btn_cancel).setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
finish();
}
});
}
}
728x90
반응형
'App > Android Studio' 카테고리의 다른 글
프로필 수정 📌미완성 (0) | 2022.08.09 |
---|---|
Android Studio - 복습 (0) | 2022.08.09 |
Android Studio - 암시적 인텐트 (0) | 2022.08.09 |
8강 이상형 월드컵 📌수정중 (0) | 2022.08.08 |
Android Studio - 명시적 인텐트 (0) | 2022.08.08 |
댓글