728x90
반응형
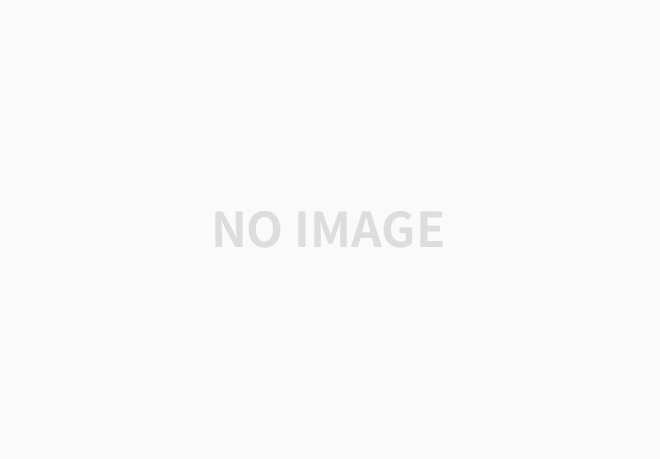
📃 Button 객체 예제(1)
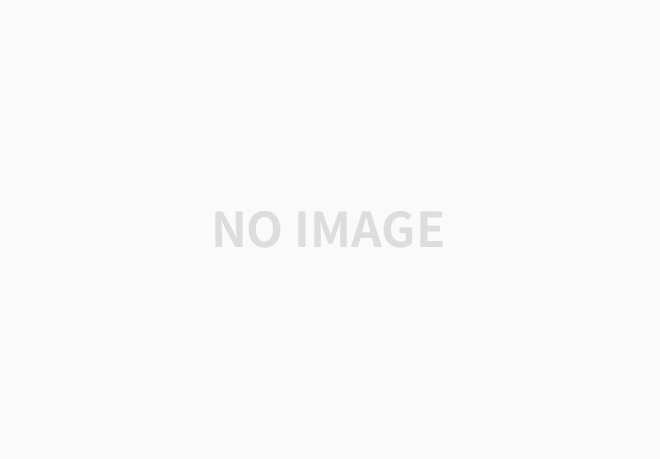
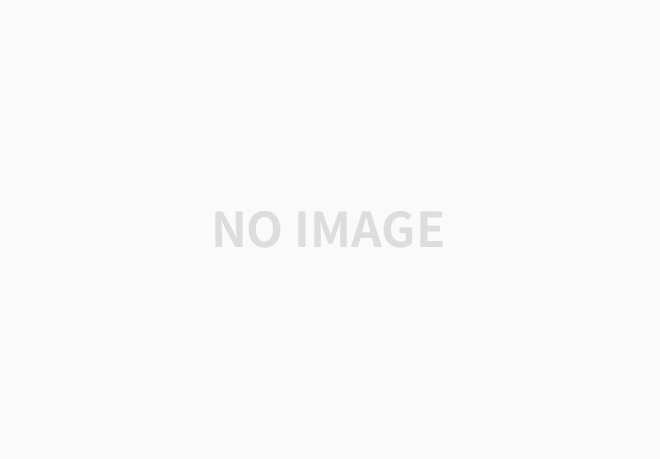
button을 매개변수로 받는 show메서드를 js로 작성한다.
버튼은 <input> 태그의 "button" 타입으로 만든다.
caption 변수에 버튼의 값을 저장하고 각각의 버튼을 누를 때마다 해당 버튼이 눌렸다는 경고창을 띄운다.
<html>
<head>
<title>java script</title>
<script type = "text/javascript">
function show(button) {
caption = button.value;
alert(caption + "가 눌려졌습니다.");
}
</script>
</head>
<body>
<h1>Button 객체 예제</h1>
다음의 단추를 누르면 단추의 종류가 나타납니다.<p>
<form name = "myForm">
<input type = "button" value = "첫번째 단추" onclick = "show(this)"/>
<input type = "button" value = "두번째 단추" onclick = "show(this)"/> <p>
<input type = "button" value = "세번째 단추" onclick = "show(this)"/>
<input type = "button" value = "네번째 단추" onclick = "show(this)"/> <p>
<input type = "button" value = "세번째 단추" onclick = "show(this)"/>
<input type = "button" value = "세번째 단추" onclick = "show(this)"/>
</form>
</body>
</html>
📃 Button 객체 예제(2)
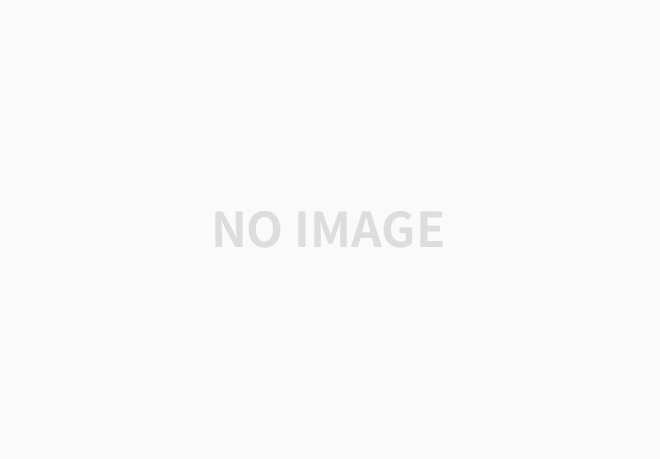
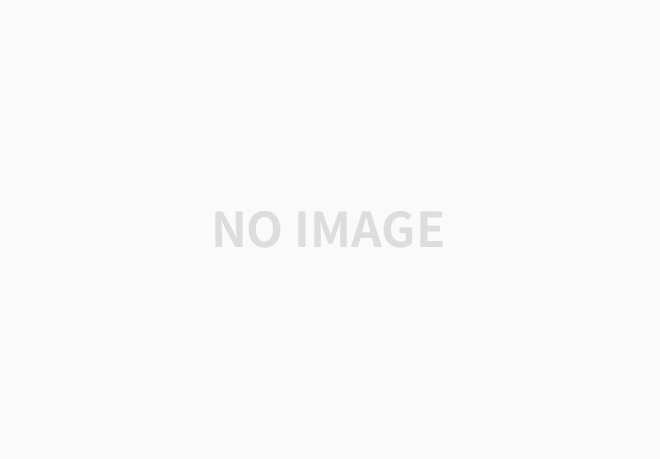
버튼을 눌렀을 때 경고창을 띄우는 show1, show2 메서드를 생성한다.
1번 단추를 누르면 1번 단추와 2번 단추를 눌렀다는 경고창이 띄워지고,
2번 단추를 누르면 2번 단추를 눌렀다는 경고창만 띄워진다.
<html>
<head>
<title>java script</title>
<script type = "text/javascript">
function show1() {
alert("1번 단추가 눌려졌습니다.");
document.myForm.myBtn2.click();
}
function show2() {
alert("2번 단추가 눌려졌습니다.");
}
</script>
</head>
<body>
<h1>Button 객체 예제</h1>
1번 단추를 누르면 2번 단추도 같이 눌려집니다.<p>
<form name = "myForm">
<input type = "button" name = "myBtn1" value = "1번 단추" OnClick = "show1()"/>
<input type = "button" name = "myBtn2" value = "2번 단추" OnClick = "show2()"/>
</form>
</body>
</html>
📃 text, textarea, password 객체 예제
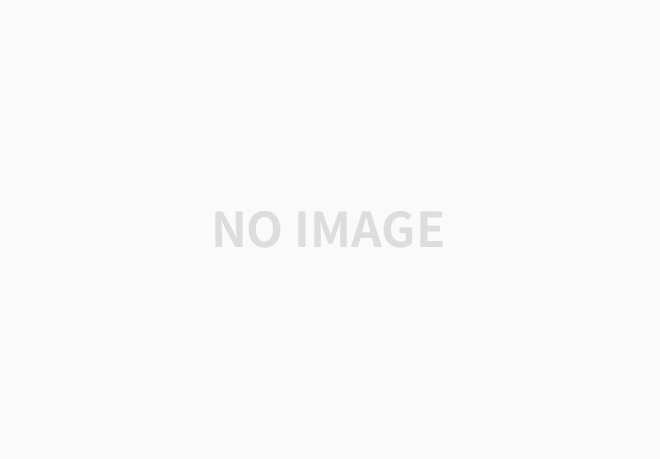
text, password 객체
속성
document.폼이름.객체이름.value ==> 현재 입력된 내용
document.폼이름.객체이름.defaultValue == > 초기값
메소드
document.폼이름.객체이름.focus(); ==> 해당 객체에 포커스를 준다.
document.폼이름.객체이름.blur(); ==> 해당 객체의 포커스를 없애준다.
document.폼이름.객체이름.select(); ==> 해당 객체의 내용을 블록지정한다.
textarea 객체
속성에 value는 없다.
나머지는 위와 동일함.
확인 버튼을 누르면 txtData인 <input> 태그에 적혀있는 값이 taData에 입력된다.
text, password, textarea태그, button으로 이루어져 있다.
<html>
<head>
<title>java script</title>
<script type = "text/javascript">
function test() {
document.testForm.taData.value += document.testForm.txtData.value + "\n";
}
</script>
</head>
<body>
<form name = "testForm">
<input type = "text" name = "txtData" value = "처음값"/><br>
<input type = "password" name = "passData"/><br>
<textarea name = "taData" rows = "30" cols = "60">초기값</textarea><br>
<input type = "button" value = "확인" OnClick = "test()"/>
</form>
</body>
</html>
📃 Text 객체 예제(1)
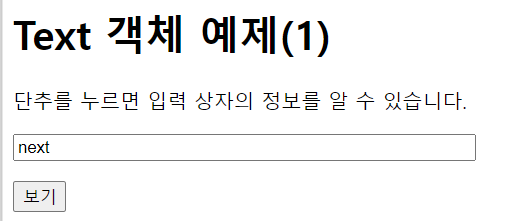
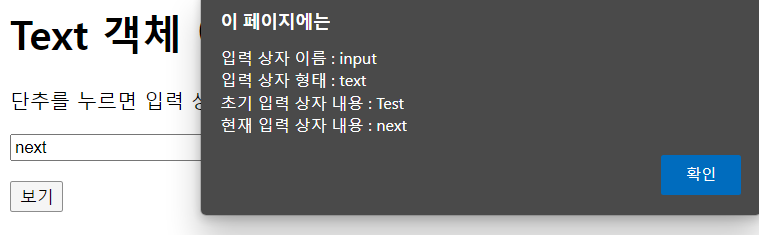
현재 입력 상자의 내용을 바꾸면 보기 버튼의 현재 입력 상자 내용도 변경된다.
보기 버튼을 누르게 되면 경고창에 입력 상자의 name과 type, 초기 입력 값과 현재 입력 값을 알 수 있다.
<html>
<head>
<title>java script</title>
<script type = "text/javascript">
function show() {
str = "입력 상자 이름 : " + document.myForm.input.name + "\n";
str += "입력 상자 형태 : " + document.myForm.input.type + "\n";
str += "초기 입력 상자 내용 : " + document.myForm.input.defaultValue + "\n";
str += "현재 입력 상자 내용 : " + document.myForm.input.value;
alert(str);
}
</script>
</head>
<body>
<h1>Text 객체 예제(1)</h1>
단추를 누르면 입력 상자의 정보를 알 수 있습니다.<p>
<form name = "myForm">
<input type = "text" name = "input" value = "Test" size = "50" maxlength = "50"><p>
<input type = "button" value = "보기" OnClick = "show()"/>
</form>
</body>
</html>
📃 Text 객체 예제(2)
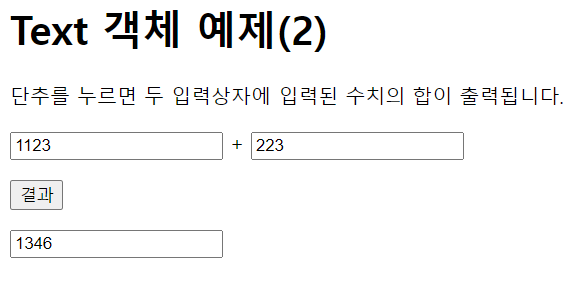
num1에 입력한 값과 num2에 입력한 값을 "결과" 버튼을 클릭할 때,
cal함수를 호출하여 myForm에 있는 num1과 num2의 합을 구하고 result <input> 태그에 text 형태로 출력한다.
<html>
<head>
<title>java script</title>
<script type = "text/javascript">
function cal() {
num1 = document.myForm.num1.value;
num2 = document.myForm.num2.value;
<!-- eval : 문자열 식 값을 반환 -->
document.myForm.result.value = (eval(num1) + eval(num2));
}
</script>
</head>
<body>
<h1>Text 객체 예제(2)</h1>
단추를 누르면 두 입력상자에 입력된 수치의 합이 출력됩니다.<p>
<form name = "myForm">
<input type = "text" name = "num1"> + <input type = "text" name = "num2"><p>
<input type = "button" value = "결과" OnClick = "cal()"><p> <!-- 결과 버튼을 누르면 cal함수 실행-->
<input type = "text" name = "result">
</form>
</body>
</html>
📃 Text 객체 예제(3)
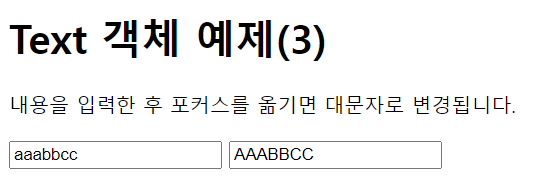
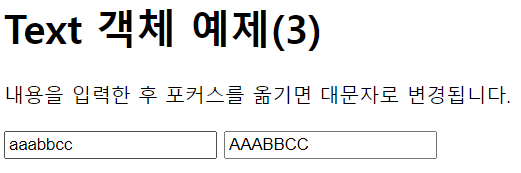
onblur는 포커스를 해제하는 event이다.
myInput이라는 이름을 가진 text타입 <input>태그에서 포커스가 해제되었을 때 upper함수를 호출하고,
upper함수의 매개변수에 입력 값을 넣는다.
upper함수에서 매개변수로 받은 값을 toUpperCase메서드를 이용하여 대문자로 모두 변환한 후,
myForm에 있는 myOutput의 값에 출력한다.
<html>
<head>
<title>java script</title>
<script type = "text/javascript">
function upper(input) {
str = input.value;
document.myForm.myOutput.value = str.toUpperCase();
}
</script>
</head>
<body>
<h1>Text 객체 예제(3)</h1>
내용을 입력한 후 포커스를 옮기면 대문자로 변경됩니다.<p>
<form name = "myForm">
<input type = "text" name = "myInput" onblur="upper(this)"/>
<input type = "text" name = "myOutput"/>
</form>
</body>
</html>
📃 Text 객체 예제(4)
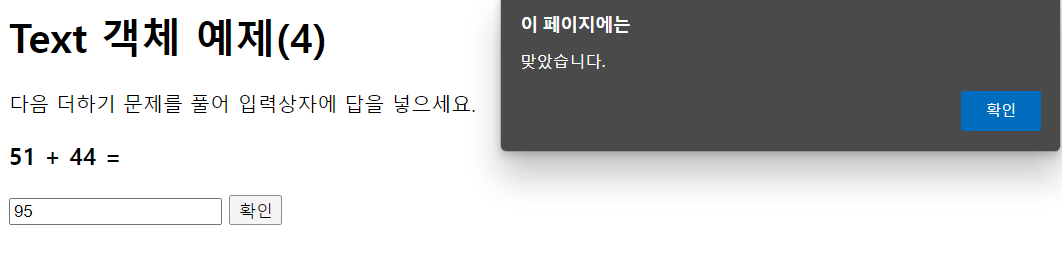
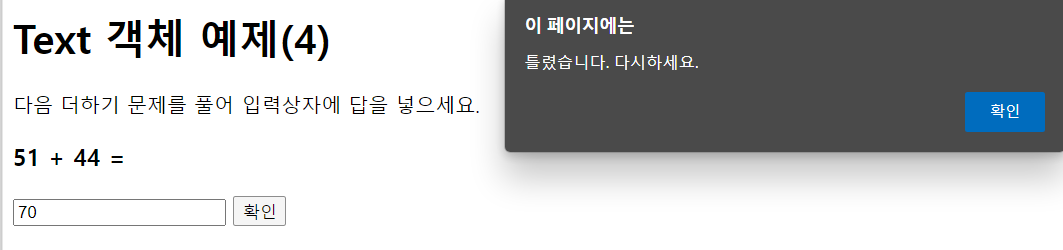
num1과 num2는 1~100까지의 값 중 랜덤 값을 선택한다.
이름이 myForm인 <form> 태그에서는 사용자가 결과 값을 입력한다.
사용자가 "확인" 버튼을 눌렀을 때 check메서드가 호출된다.
check메서드는 myDap과 yourDap의 지역변수를 가진다.
myDap에는 랜덤 값 두개의 합을 저장하고, yourDap에는 사용자가 입력한 값을 저장한다.
만약 myDap과 yourDap이 맞는다면 맞았다는 경고창을 띄우고, 틀렸다면 틀렸다는 경고창을 띄운다.
각각 경고창을 띄우고 난 후 값에는 포커스를 주었다가(focus()), 해제(blur())한다.
💬 Math.floor() 함수는 주어진 숫자와 같거나 작은 정수 중에서 가장 큰 수를 반환한다.
<html>
<head>
<title>java script</title>
<script type = "text/javascript">
function start() {
document.myForm.result.focus();
}
function check() {
myDap = num1 + num2;
yourDap = document.myForm.result.value;
if(myDap == yourDap) {
alert("맞았습니다.");
document.myForm.result.focus();
document.myForm.result.blur();
}
else {
alert("틀렸습니다. 다시하세요.");
document.myForm.result.focus();
document.myForm.result.blur();
}
}
</script>
</head>
<body>
<h1>Text 객체 예제(4)</h1>
다음 더하기 문제를 풀어 입력상자에 답을 넣으세요.<p>
<script type = "text/javascript">
num1 = Math.floor(Math.random()*100 + 1);
num2 = Math.floor(Math.random()*100 + 1);
document.write("<h3>" + num1 + " + " + num2 + " =</h3>");
</script>
<form name = "myForm">
<input type = "text" name = "result">
<input type = "button" value = "확인" onclick = "check()"
</form>
</body>
</html>
📃 구구단
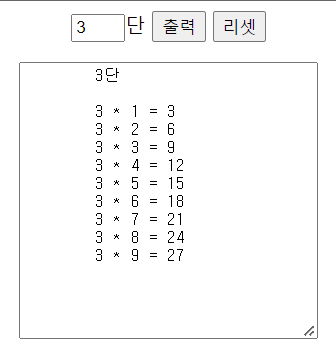
<center> 태그를 통해 <form> 태그의 내용을 가운데로 놓는다.
<form> 태그 안에 size가 1이고 maxlength 속성을 통해 최대 입력할 수 있는 문자를 1개로 고정시킨다. 이 태그의 이름은 dan이다.
"출력" 버튼을 누르면 gugu메서드를 실행하고 "리셋" 버튼을 누르면 reset될 수 있도록 type속성을 "reset"으로 바꾼다.
구구단이 출력되는 <textarea> 태그는 행 15, 열 30으로 사이즈를 맞춘다.
gugu함수는 변수 f를 통해 myForm 태그를 저장하고, dan에는 myForm 태그 안에 있는 dan의 값을 가져온다.
dan*1의 값이 dan값이거나 dan이 비었거나 dan이 숫자 값이 아니라면 숫자를 입력하라는 경고창이 띄워진다.
그렇지 않다면, myForm 태그 안에 있는 area의 값에 *(1~9) 를 시행하여 구구단을 출력한다.
💬 isNaN() 함수 : 매개변수가 숫자가 아닌 값을 찾는 함수
<html>
<head>
<title>java script</title>
<script type = "text/javascript">
function gugu() {
var f = document.myForm;
var dan = f.dan.value;
if(dan * 1 != dan || dan == "" || isNaN(dan)) {
alert("숫자를 입력하세요");
}
else {
f.area.value = "\t" + dan + "단\n\n"
for(i = 1; i<10; i++) {
f.area.value += "\t" + dan + " * " + i + " = " + (dan*i) + "\n";
}
}
}
</script>
</head>
<body>
<center>
<form name = "myForm">
<input type = "text" size = "1" maxlength = "1" name = "dan"/>단
<input type = "button" value = "출력" onClick="gugu()"/>
<input type = "reset" value = "리셋"/><p>
<textarea name = "area" rows = "15" cols = "30"/></textarea>
</form>
</center>
</body>
</html>
📃 Radio, Checkbox 예제
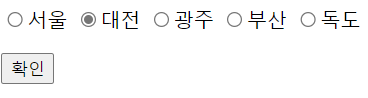
<form> 태그의 myForm에 type이 "radio"속성을 가진 "mychk"를 "서울", "대전", "광주", "부산", "독도" 만든다.
대전은 checked 속성을 주어 체크되었는지 안됐는지 확인할 수 있다.
또한, <form> 태그의 myForm에 type이 "button"이고 "확인"이 적혀있는 버튼을 만든다.
"확인" 버튼을 누르면 view함수가 호출된다.
view함수는 f 변수에 myForm에 있는 "mychk"이름인 "radio"들을 저장한다.
checkbox, radio 객체 ( 다 똑같은데 차이는 checkbox는 중복허용가능, radio는 중복불가 )
- 객체 이름이 동일한 checkbox(radio)가 1개일 경우
document.폼이름.객체이름.value ==> 해당 객체의 value값
document.폼이름.객체이름.checked ==> 해당 객체의 현재 check상태를 나타낸다. (true,false)
(check상태 : true, 미 check상태 : false)
document.폼이름.객체이름.defaultChecked ==> 초기의 check 상태를 나타낸다. (true,false)
(check상태 : true, 미 check상태 : false)
- 객체 이름이 동일한 checkbox(radio)가 여러 개일 경우
(객체이름의 배열에 checkbox가 저장된다.)
document.폼이름.객체이름.length ==> '객체이름'으로 되어있는 checkbox개수
document.폼이름.객체이름[첨자].value
document.폼이름.객체이름[첨자].checked ==> 의미는 위와 동일함.
document.폼이름.객체이름[첨자].defaultChecked ==> 의미는 위와 동일함.
- 메소드
(한개일 경우)
document.폼이름.객체이름.click(); // 말그대로 해당객체를 클릭해준다.
(여러개일 경우)
document.폼이름.객체이름[첨자].click(); // 똑같이 해당각체를 클릭해준다.
📃 Check 객체 예제(1)

<input> 태그 안에 "checkbox"속성을 가지고 이름이 "check"인 "Yahoo" 체크 버튼과
"button"속성을 가지고 "보기"라고 적혀있는 버튼을 만든다.
"보기" 버튼을 클릭하면 Show함수를 호출한다.
Show함수는 경고창을 띄워주며, 경고창에는 체크 상자의 형태, 이름, 값을 출력하도록 세팅한다.
<html>
<head>
<title>java script</title>
<script type = "text/javascript">
function Show() {
str = "체크 상자의 형태 : " + document.myForm.check.type + "\n";
str += "체크 상자의 이름 : " + document.myForm.check.name + "\n";
str += "체크 상자의 값 : " + document.myForm.check.value;
alert(str);
}
</script>
</head>
<body>
<h3>Check 객체 예제(1)</h3>
다음 단추를 누르면 체크 상자에 대한 정보를 알 수 있습니다.<p>
<form name = "myForm">
<input type = "checkbox" value = "Yahoo" name = "check">야후<p>
<input type = "button" value = "보기" onclick = "Show()">
</form>
</body>
</html>
📃 Check 객체 예제(2)
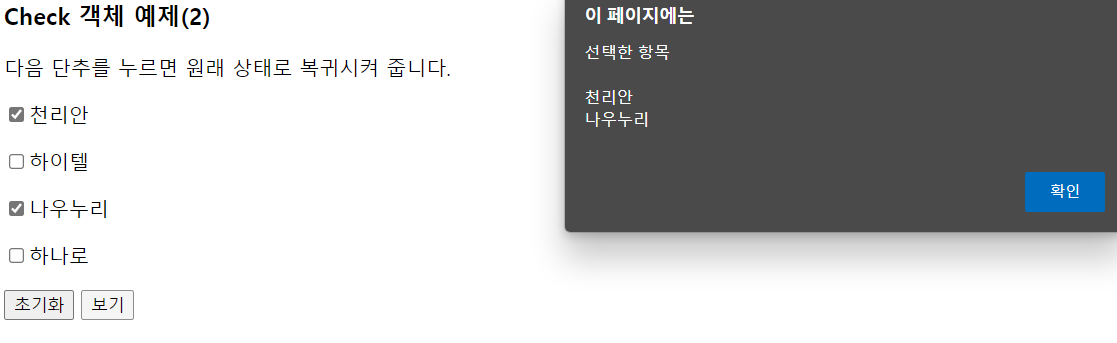
<input> 태그 안에 "checkbox" 속성을 가지고 이름이 "check"인 체크 박스를 4개 생성하고,
각각 "천리안", "하이텔", "나우누리", "하나로" 값을 준다.
또한, "button"속성을 가지는 버튼을 "초기화"와 "보기" 두개 만든다.
"초기화" 버튼을 누르면 this.form을 매개변수로 가지는 Change함수를 호출하고,
"보기" 버튼을 누르면 this.form을 매개변수로 가지는 show함수를 호출한다.
form을 매개변수로 가지는 Change함수는 "check"가 체크되었을 때 true값을 반환하고,
그 외는 모두 false로 반환한다.
form을 매개변수로 가지는 show함수는 select 지역변수를 "off"로 설정하고, str 지역변수를 "선택한 항목"이라는 문자열로 설정한다.
만약 "check"가 체크되었다면 체크된 항목만 str변수에 넣고 select 변수를 "on"으로 설정한다.
만약 select 변수가 "on"이라면 alert 경고창을 이용하여 str변수에 저장된 값을 모두 보여준다.
그 외라면 선택한 항목이 없다고 경고창을 띄워준다.
<html>
<head>
<title>java script</title>
<script type = "text/javascript">
function Change(form) {
for(i=0; i<4; i++) {
if(form.check[i].defaultChecked == true) {
form.check[i].checked = true;
}
else {
form.check[i].checked = false;
}
}
}
function show(form) {
var select = "off";
var str = "선택한 항목 \n\n";
for(i=0; i<4; i++) {
if(form.check[i].checked == true) {
str += form.check[i].value + "\n";
select = "on";
}
}
if(select == "on") {
alert(str);
}
else {
alert("선택한 항목이 없습니다.");
}
}
</script>
</head>
<body>
<h3>Check 객체 예제(2)</h3>
다음 단추를 누르면 원래 상태로 복귀시켜 줍니다.<p>
<form name = "myForm">
<input type = "checkbox" name = "check" value = "천리안" >천리안<p>
<input type = "checkbox" name = "check" value = "하이텔" >하이텔<p>
<input type = "checkbox" name = "check" value = "나우누리" >나우누리<p>
<input type = "checkbox" name = "check" value = "하나로" >하나로<p>
<input type = "button" value = "초기화" onclick = "Change(this.form)">
<input type = "button" value = "보기" onclick = "show(this.form)">
</form>
</body>
</html>
📃 Radio 객체 예제(1)
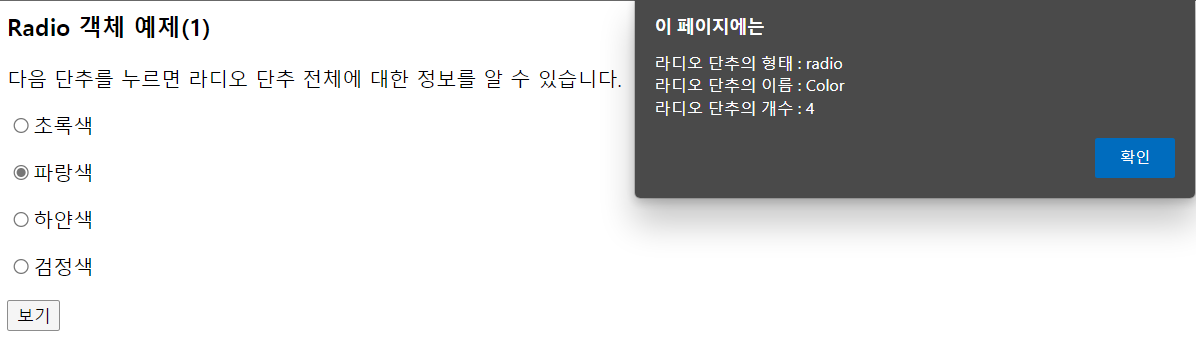
<input> 태그 안에 "radio" 속성을 가지고 이름이 "Color"인 라디오 버튼 4개를 생성하고,
각각의 label은 초록색, 파랑색, 하얀색, 검정색이다.
"button"속성을 가지고 값이 "보기"인 버튼을 생성하고, 클릭했을 때 show함수를 호출한다.
show함수는 alert 경고창을 이용하여 str의 값을 출력한다.
str는 라디오 단추의 형태, 이름, 개수가 들어가있다.
형태는 myForm에 있는 "Color"의 type 출력
이름은 myForm에 있는 "Color"의 name 출력
개수는 myForm에 있는 "Color"의 length를 출력한다.
<html>
<head>
<title>java script</title>
<script type = "text/javascript">
function show() {
str = "라디오 단추의 형태 : " + document.myForm.Color[0].type + "\n";
str += "라디오 단추의 이름 : " + document.myForm.Color[0].name + "\n";
str += "라디오 단추의 개수 : " + document.myForm.Color.length;
alert(str);
}
</script>
</head>
<body>
<h3>Radio 객체 예제(1)</h3>
다음 단추를 누르면 라디오 단추 전체에 대한 정보를 알 수 있습니다.<p>
<form name = "myForm">
<input type = "radio" name = "Color">초록색<p>
<input type = "radio" name = "Color">파랑색<p>
<input type = "radio" name = "Color">하얀색<p>
<input type = "radio" name = "Color">검정색<p>
<input type = "button" value = "보기" onclick="show()">
</form>
</body>
</html>
📃 Radio 객체 예제(2)
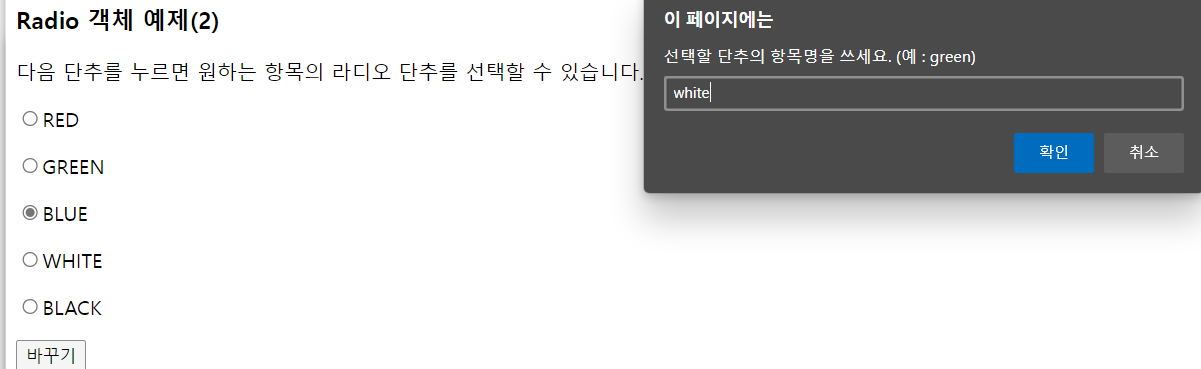
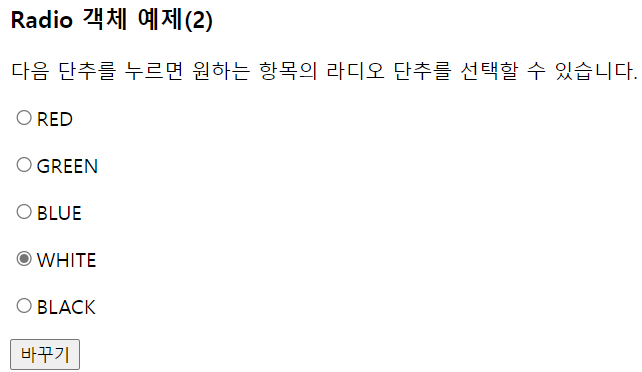
<input> 태그 안에 "radio" 속성을 가지고 이름이 "Color"인 라디오 버튼 5개를 생성하고,
각각의 값은 "RED", "GREEN", "BLUE", "WHITE", "BLACK"이다.
"button"속성을 가지고 값이 "바꾸기"인 버튼을 생성하고, 클릭했을 때 매개변수가 this.form인 Change함수를 호출한다.
form을 매개변수로 가지는 Change함수는 select가 "no"인 변수를 생성하고,
사용자에게 값을 입력받는 str를 가진다.
사용자가 값을 입력하면 값을 모두 대문자로 바꾸고, 체크 표시를 true로 바꾼 후, select를 "ok"로 변경한다.
만약 select가 "no"라면 항목에 없다고 경고창으로 알려준다.
<html>
<head>
<title>java script</title>
<script type = "text/javascript">
function Change(form) {
var select = "no";
str = prompt("선택할 단추의 항목명을 쓰세요. (예 : green)", "");
for(i=0; i<form.Color.length; i++) {
if(form.Color[i].value == str.toUpperCase()) {
form.Color[i].checked = true;
select = "ok";
}
}
if(select == "no") {
alert("항목에 없습니다.");
}
}
</script>
</head>
<body>
<h3>Radio 객체 예제(2)</h3>
다음 단추를 누르면 원하는 항목의 라디오 단추를 선택할 수 있습니다.<p>
<form>
<input type = "radio" name = "Color" value = "RED">RED<p>
<input type = "radio" name = "Color" value = "GREEN">GREEN<p>
<input type = "radio" name = "Color" value = "BLUE">BLUE<p>
<input type = "radio" name = "Color" value = "WHITE">WHITE<p>
<input type = "radio" name = "Color" value = "BLACK">BLACK<p>
<input type = "button" value = "바꾸기" onclick="Change(this.form)">
</form>
</body>
</html>
📃 Radio 객체 예제(3)
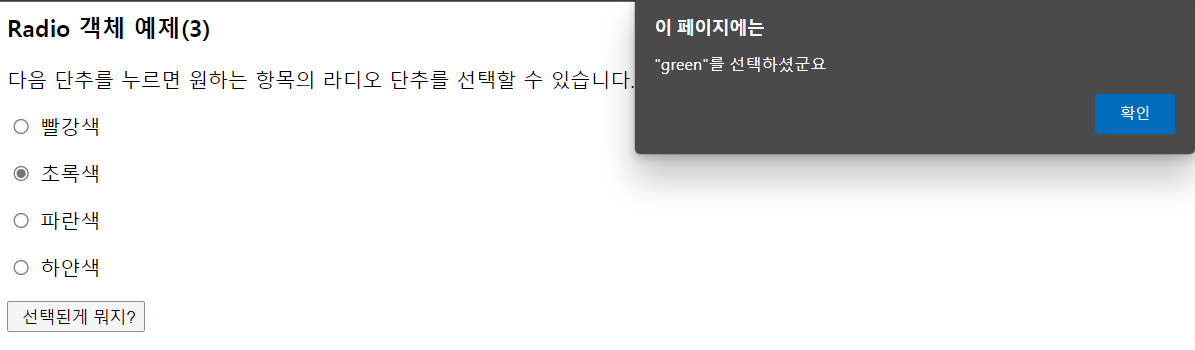

<input> 태그 안에 "radio" 속성을 가지고 이름이 "Color"인 라디오 버튼 4개를 생성하고,
각각의 값은 "red", "green", "blue", "WHITE"이다.
"button"속성을 가지고 값이 "선택된게 뭐지?"인 버튼을 생성하고, 클릭했을 때 매개변수가 this.form인 Change함수를 호출한다.
form을 매개변수로 가지는 Change함수는 체크박스가 체크되었다면,
선택한 색상을 알려주는 경고창을 표시하고 바탕색을 체크된 색상의 값으로 변경한다.
<html>
<head>
<title>java script</title>
<script type = "text/javascript">
function Change(form) {
for(i=0; i<form.Color.length; i++) {
if(form.Color[i].checked) {
document.bgColor = form.Color[i].value;
alert("\"" + form.Color[i].value + "\"를 선택하셨군요");
}
}
}
</script>
</head>
<body>
<h3>Radio 객체 예제(3)</h3>
다음 단추를 누르면 원하는 항목의 라디오 단추를 선택할 수 있습니다.<p>
<form>
<input type= "radio" value = "red" name = "Color" checked> 빨강색 <p>
<input type= "radio" value = "green" name = "Color" > 초록색 <p>
<input type= "radio" value = "blue" name = "Color" > 파란색 <p>
<input type= "radio" value = "white" name = "Color" > 하얀색 <p>
<input type= "button" value = " 선택된게 뭐지?" onClick = "Change(this.form)" >
</form>
</body>
</html>
📃 Select 객체 예제(1)
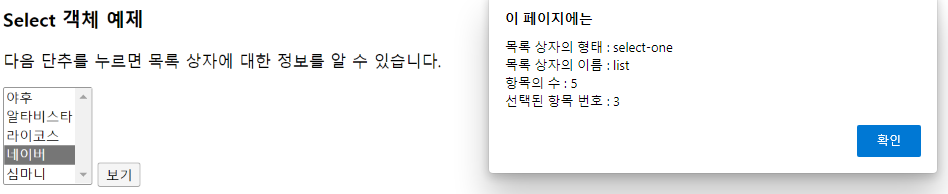
<form> 태그 안에 <select> 태그를 넣어 선택할 수 있는 옵션을 넣는다.
옵션은 총 5개로, 각각의 옵션은 야후, 알타비스타, 라이코스, 네이버, 심마니로 이루어져있다.
<input> 태그 안에는 "button"속성이 있으며 "보기" 라고 적혀있고, 클릭할 때 show함수가 호출된다.
show함수는 alert를 이용하여 경고창을 띄워주고 매개변수는 str이다.
str안에는 목록 상자의 형태, 이름, 항목의 수, 선택된 항목 번호가 나와있다.
선택된 항목 번호는 selectedIndex로 표시할 수 있고, 0부터 시작이다.
<html>
<head>
<title>java script</title>
<script type = "text/javascript">
function show() {
str = "목록 상자의 형태 : " + document.myForm.list.type + "\n";
str += "목록 상자의 이름 : " + document.myForm.list.name + "\n";
str += "항목의 수 : " + document.myForm.list.length + "\n";
str += "선택된 항목 번호 : " + document.myForm.list.selectedIndex;
alert(str);
}
</script>
</head>
<body>
<h3>Select 객체 예제</h3>
다음 단추를 누르면 목록 상자에 대한 정보를 알 수 있습니다.<p>
<form name = "myForm">
<select name = "list" size = "5">
<option>야후</option>
<option>알타비스타</option>
<option>라이코스</option>
<option>네이버</option>
<option>심마니</option>
</select>
<input type = "button" value = "보기" onclick="show()">
</form>
</body>
</html>
📃 Select 객체 예제(2)
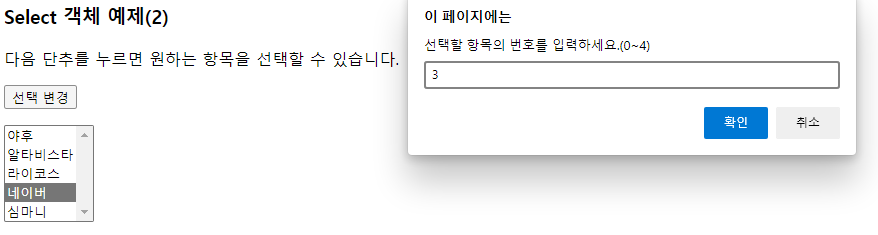
<form> 태그 안에 옵션을 선택할 수 있는 <select> 태그가 있고, size는 5이며, multiple 옵션으로 모든 옵션을 한 번에 볼 수 있다.
옵션은 야후, 알타비스타, 라이코스, 네이버, 심마니로 이루어져 있다.
<input> 태그에 있는 "button"속성을 가지고 있고 "선택 변경"으로 적혀있는 버튼은 눌렀을 때 Select함수를 호출한다.
Select함수는 num 변수에 사용자가 입력하는 번호의 값을 넣어서 그 번호의 인덱스를 가져온다.
<html>
<head>
<title>java script</title>
<script type = "text/javascript">
function Select() {
num = prompt("선택할 항목의 번호를 입력하세요.(0~4)", "");
document.myForm.list.selectIndex = num;
}
</script>
</head>
<body>
<h3>Select 객체 예제(2)</h3>
다음 단추를 누르면 원하는 항목을 선택할 수 있습니다.<p>
<form name = "myForm">
<input type = "button" value = "선택 변경" onclick="Select()"><p>
<select name = "list" size = "5" multiple>
<option>야후</option>
<option>알타비스타</option>
<option>라이코스</option>
<option>네이버</option>
<option>심마니</option>
</select>
</form>
</body>
</html>
📃 Select 객체 예제(3)
select 객체
1. 콤보박스 (size를 생략하거나 size값이 1일경우)
2. 리스트박스 (size 가 2 이상일 경우)
multiple ==> 컨트롤키 누르고 여러개 클릭하면 여러개 선택 가능하게 해줌
select 객체의 속성
document.폼이름.객체이름.length ==> 항목수 반환해줌.
document.폼이름.객체이름.selectedIndex ==> 현재 선택된 항목의 index번호를 이야기함.
( index번호는 0번부터 시작한다. )
( 선택항목이 없을경우에는 : -1 을반환한다. )
-- 각 항목의 대한 속성들
document.폼이름.객체이름.option[첨자].value ==> 첨자번째의 value값
document.폼이름.객체이름.option[첨자].text ==> 첨자번째의 text값
document.폼이름.객체이름.option[첨자].selected ==> 첨자번째의 선택 여부
(선택 상태 : true, 미 선택 상태 : false);
document.폼이름.객체이름.option[첨자].defaultSelected ==> 첨자번째의 선택 여부
(초기 선택 : true, 초기 미 선택 : false);
-- 런타임시 새로운 항목 추가 및 삭제
1. 추가
var 변수 = new Option(text값, value값);
document.폼이름.객체이름.options[추가할 위치] = 변수;
2. 삭제
document.폼이름.객체이름.options[삭제할 위치] = null;
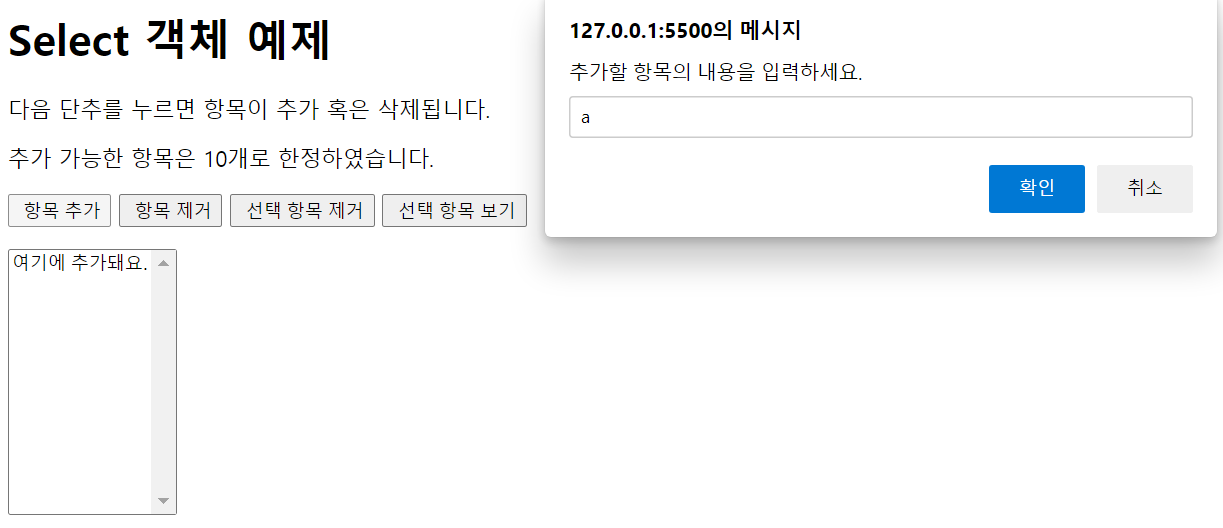
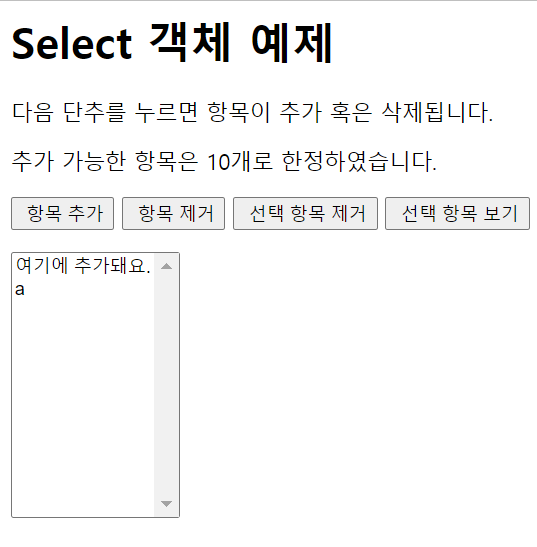
<input> 태그의 "button"속성을 가지는 버튼을 4개 만들고,
각각 항목 추가, 항목 제거, 선택 항목 제거, 선택 항목 보기로 이루어져있다.
항목 추가 버튼을 눌렀을 때, Append 함수가 호출된다.
Append 함수는 prompt를 통해 추가할 항목의 내용을 입력하고 저장한다.
크기가 10보다 클 경우 더이상 추가할 수 없다는 경고문을 띄운다.
항목 제거 버튼을 눌렀을 때, Delete 함수가 호출된다.
Delete 함수는 prompt를 통해 추가할 항목의 내용을 입력하고 저장한다.
길이가 0일 경우 더이상 삭제할 자료가 없다는 경고문을 띄운다.
선택 항목 제거 버튼을 눌렀을 때, Select_Delete 함수가 호출된다.
Select_Delete 함수는 선택한 인덱스의 번호의 문자열을 null로 바꾼다.
길이가 0일 경우 더이상 삭제할 자료가 없다는 경고문을 띄우고,
인덱스가 -1일 경우는 선택 항목이 없다는 경고문을 띄운다.
선택 항목 보기 버튼을 눌렀을 때, show 함수가 호출된다.
show 함수는 선택된 항목의 인덱스와 내용을 alert 경고문을 통해 띄운다.
인덱스가 0보다 작을 경우는 선택 항목이 없다고 경고문을 띄운다.
<html>
<head>
<title> JAVA SCRIPT </title>
<script type= "text/javascript">
function Append(){
loc = document.myForm.list.length;
if(loc >= 10){
alert("더이상 추가할 수 없습니다.");
return;
}
str = prompt("추가할 항목의 내용을 입력하세요.","");
myOption = new Option(str);
document.myForm.list.options[loc] = myOption;
}
function Delete(){
loc = document.myForm.list.length;
if(loc == 0){
alert("더이상 삭제할 자료가 없습니다.");
return;
}
document.myForm.list.options[loc-1] = null;
}
function Select_Delete(){
num = document.myForm.list.selectedIndex;
loc = document.myForm.list.length;
if(num == -1){
alert("선택 항목이 없습니다.");
return;
}
if(loc == 0){
alert("더이상 삭제할 자료가 없습니다.");
return;
}
document.myForm.list.options[num] = null;
}
function show(form){
num = form.list.selectedIndex;
if(num < 0){
alert("선택 항목이 없습니다.");
return;
}
str = "선택된 항목의 인덱스 : " + form.list.options[num].index + "\n";
str += "선택된 항목의 내용 : " + form.list.options[num].text;
alert(str);
}
</script>
</head>
<body>
<h1>Select 객체 예제</h1>
다음 단추를 누르면 항목이 추가 혹은 삭제됩니다.<p>
추가 가능한 항목은 10개로 한정하였습니다.<p>
<form name = "myForm">
<input type= "button" value = " 항목 추가" onClick="Append()">
<input type= "button" value = " 항목 제거" onClick="Delete()">
<input type= "button" value = " 선택 항목 제거" onClick="Select_Delete()">
<input type= "button" value = " 선택 항목 보기" onClick="show(this.form)"><p>
<select name = "list" size = "10">
<option>여기에 추가돼요.</option>
</select>
</form>
</body>
</HTML>
📃 Select 객체 예제(4)
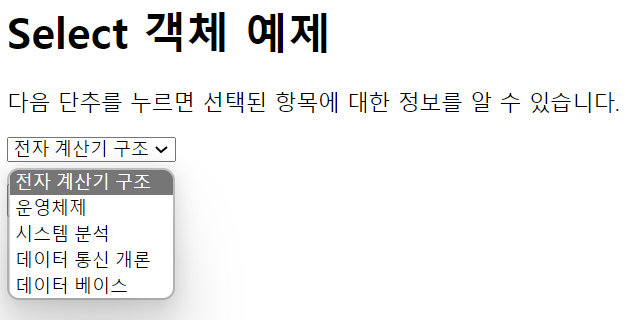
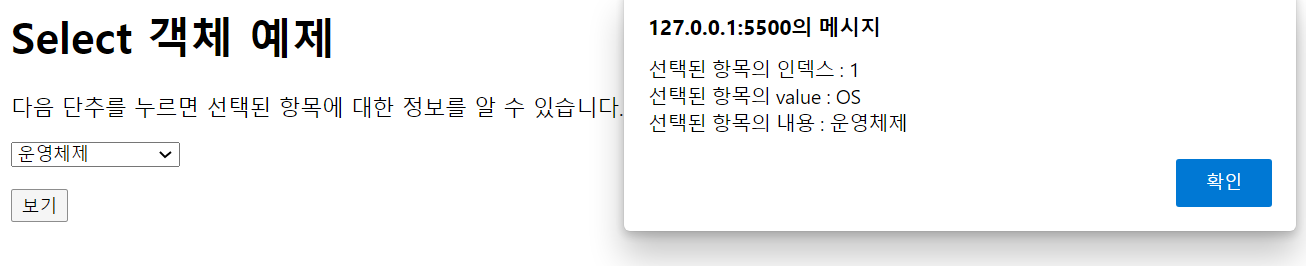
<form> 태그 안에 <select> 태그를 넣고 그 안에 <option>태그를 5개 넣는다.
<option> 태그의 각 요소는 전자 계산기 구조, 운영체제, 시스템 분석, 데이터 통신 개론, 데이터 베이스이다.
<input> 태그 "button"속성의 "보기" 버튼은 누르면 this.form를 매개변수로 갖는 show함수를 호출한다.
show 함수는 str를 경고창으로 띄운다.
str는 선택된 항목의 인덱스, value, 내용을 포함한다.
인덱스가 0보다 작으면 선택 항목이 없다고 경고창을 띄운다.
<html>
<head>
<title> JAVA SCRIPT </title>
<script type= "text/javascript">
function show(form){
num = form.combo.selectedIndex;
if(num < 0){
alert("선택 항목이 없습니다.");
return;
}
str = "선택된 항목의 인덱스 : " + form.combo.options[num].index + "\n";
str += "선택된 항목의 value : " + form.combo.options[num].value + "\n";
str += "선택된 항목의 내용 : " + form.combo.options[num].text;
alert(str);
}
</script>
</head>
<body>
<h1>Select 객체 예제</h1>
다음 단추를 누르면 선택된 항목에 대한 정보를 알 수 있습니다.<p>
<form name = "myForm">
<select name = "combo" size = "1">
<option value = "Computer">전자 계산기 구조</option>
<option value = "OS">운영체제</option>
<option value = "System">시스템 분석</option>
<option value = "Data">데이터 통신 개론</option>
<option value = "DB">데이터 베이스</option>
</select><p>
<input type= "button" value = "보기" onClick="show(this.form)">
</form>
</BODY>
</html>
📃Frame 객체 예제(1)
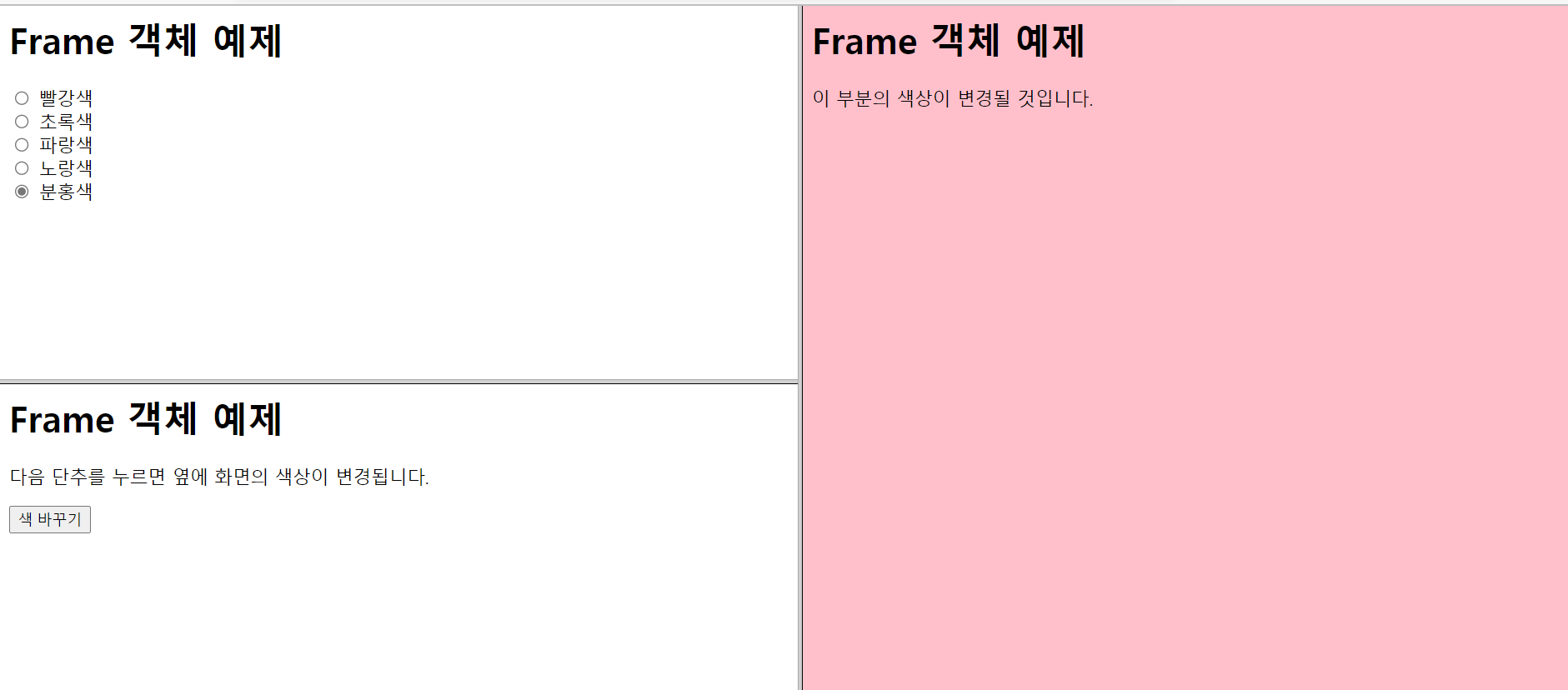
📝 JS.html
<frameset> 태그를 이용해 <frame> 을 3개를 만든다.
각각 <frame>은 [📝 sub_2_1.html ], [📝 sub_2_2.html ], [📝 sub_2_3.html ] 를 연결한다.
<html>
<head>
<title> JAVA SCRIPT </title>
</head>
<frameset cols = "50%,50%">
<frameset rows = "50%, 50%">
<frame src = "sub_2_1.html" name = "Up">
<frame src = "sub_2_2.html" name = "Down">
</frameset>
<frame src = "sub_2_3.html" name = "Right">
</frameset>
</html>
📝 sub_2_1.html
<input> 태그를 이용해 "radio" 속성을 가지고 name이 "color"인 라디오 버튼을 5개 만든다.
각각의 라디오 버튼은 빨간색, 초록색, 파랑색, 노랑색, 분홍색을 가진다.
color_Change 함수를 가진다.
color_Change 함수는 "color"의 버튼이 체크되었을 때(true) Color에 누른 번호의 값을 넣는다.
화면의 오른쪽 프레임의 바탕색을 Color로 바꾼다.
<html>
<head>
<title> JAVA SCRIPT </title>
<script type= "text/javascript">
function color_Change(){
for(i = 0; i < document.myForm.color.length; i++){
if (document.myForm.color[i].checked == true){
Color = document.myForm.color[i].value;
}
}
top.Right.document.bgColor = Color;
}
</script>
</head>
<body>
<h1>Frame 객체 예제</h1>
<form name = "myForm">
<input type= "radio" value = "red" name = "color"> 빨강색<br>
<input type= "radio" value = "green" name = "color"> 초록색<br>
<input type= "radio" value = "blue" name = "color"> 파랑색<br>
<input type= "radio" value = "yellow" name = "color"> 노랑색<br>
<input type= "radio" value = "pink" name = "color"> 분홍색<br>
</form>
</body>
</ht>
📝 sub_2_2.html
<input> 태그를 통해 "button"속성을 가진 "색 바꾸기" 버튼을 만들고,
클릭할 때 Change 함수를 호출한다.
Change 함수는 위의 프레임이 가지고 있는 color_Change 함수를 호출한다.
<html>
<head>
<title> JAVA SCRIPT </title>
<script type= "text/javascript">
function Change(){
parent.Up.color_Change();
}
</script>
</head>
<body>
<h1>Frame 객체 예제</h1>
다음 단추를 누르면 옆에 화면의 색상이 변경됩니다.<p>
<form name= "myForm">
<input type = "button" value = "색 바꾸기" onClick="Change()">
</form>
</BODY>
</html>
📝 sub_2_3.html
<h1> 태그를 통해 글씨를 출력한다.
<html>
<head>
<title> JAVA SCRIPT </title>
</head>
<body>
<h1>Frame 객체 예제</h1>
이 부분의 색상이 변경될 것입니다.
</body>
</ht>
</html>
📃 Frame 객체 예제(2)
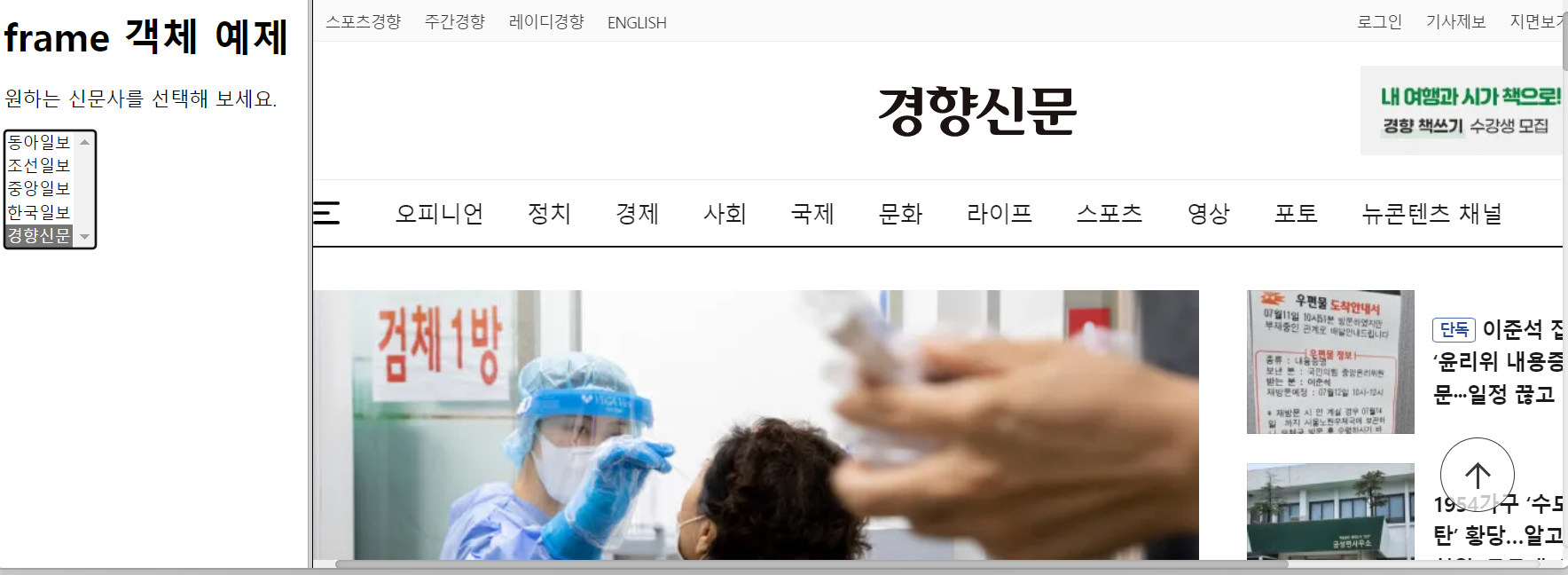
📝 main.html
<frameset> 크기를 20%, 80%으로 나눈다.
<frame> 은 두개이며 각각 [📝 sub_3-1.html ] [📝 sub_3-2.html ]이다.
[📝 sub_3-1.html ] 의 이름은 Left이고,
[📝 sub_3-2.html ] 의 이름은 Right이다.
<html>
<head>
<title> JAVA SCRIPT </title>
</head>
<frameset cols = "20%,80%">
<frame src = "sub_3-1.html" name = "Left">
<frame src = "sub_3-2.html" name = "Right">
</frameset>
</html>
📝 sub_3-1.html
<form> 태그 안에 size가 5이고 onChange event를 사용하는 <select> 태그를 사용한다.
<select> 태그 안에 동아일보, 조선일보, 중앙일보, 한국일보, 경향신문 총 5개의 <option>을 놓는다.
onChange event가 발생했을 때는 this를 매개변수로 받는 Link함수를 호출한다.
Link함수는 오른쪽에 있는 프레임의 url을 누른 옵션의 인덱스로 바꾼다.
<html>
<head>
<title>JAVA SCRIPT</title>
<script type="text/javascript">
function Link(myOption) {
index = myOption.selectedIndex;
url = myOption.options[index].value;
parent.Right.location.replace(url);
}
</script>
</head>
<body>
<h1>frame 객체 예제</h1>
원하는 신문사를 선택해 보세요.
<p></p>
<form name="myForm">
<select name="news" size="5" onChange="Link(this)">
<option value="http://www.donga.com">동아일보</option>
<option value="http://www.chosun.com">조선일보</option>
<option value="http://www.jungang.co.kr">중앙일보</option>
<option value="http://www.korealink.co.kr">한국일보</option>
<option value="http://www.khan.co.kr">경향신문</option>
</select>
</form>
</body>
</html>
📝 sub_3-2.html
<h1> 태그를 이용해서 Frame 객체 예제를 출력한다.
<html>
<head>
<title> JAVA SCRIPT </tit>
</head>
<body>
<h1>Frame 객체 예제</h1>
</body>
</ht>
📃 layer 객체 예제(1)

<HTML>
<HEAD>
<TITLE> JAVA SCRIPT </TITLE>
<script type= "text/javascript">
function showHide(){
if(document.all["myLayer"].style.visibility == "visible"){
document.all["myLayer"].style.visibility = "hidden";
}else{
document.all.myLayer.style.visibility = "visible";
}
}
</script>
</HEAD>
<BODY>
<div id = "myLayer" style = "position : absolute ; visibility : visible; background-color : #00ff00;">
이것은 레이어 안의 문자열입니다.!!!
</div>
<br><p><p>
<form name = "myForm">
<input type= "button" value = "레이어 표시하기/감추기" onClick = "showHide()">
</form>
</BODY>
</HTML>
📃 layer 실습 예제(2)
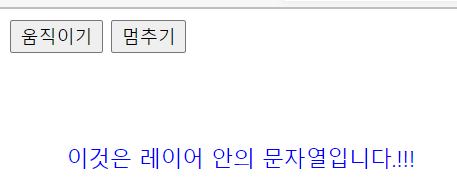
<HTML>
<HEAD>
<TITLE> JAVA SCRIPT </TITLE>
<script type= "text/javascript">
var pos = 50;
var direction = true;
var Tid;
function move(){
if(pos < 50 )direction = true;
if(pos > 250)direction = false;
if(direction)pos++;
else pos--;
document.all.myLayer.style.left = pos;
}
function moveStart(){
Tid = setInterval('move()',20);
}
function moveStop(){
clearInterval(Tid);
}
</script>
</HEAD>
<BODY>
<form name = "myForm">
<input type= "button" value = "움직이기" onClick = "moveStart()" >
<input type= "button" value = "멈추기" onClick = "moveStop()" >
</form>
<br>
<div id = "myLayer" style="position : absolute ; visibility : visible;
left : 50; top : 100; width : 500; height : 20;">
<font color = "blue"> 이것은 레이어 안의 문자열입니다.!!!</font>
</div>
</BODY>
</HTML>
728x90
반응형
'Front-End > JavaScript' 카테고리의 다른 글
JavaScript - JQuery (2) (0) | 2022.07.29 |
---|---|
JavaScript - JQuery (0) | 2022.07.28 |
JavaScript - 기본 문법 (0) | 2022.07.11 |
댓글